TypeScript Tip of the Week — Nullish Coalescing vs Logical OR Operator
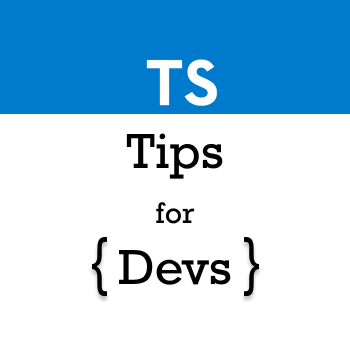
Nullish coalescing is an awesome new feature of TypeScript v 3.7. So what is nullish coalescing? Let’s look at an example.
const name = person.firstName ?? 'John';
If there is no person.firstName
then we will fall back to the value of John. Another way we could write the above code is like this.
let name;
if(person.firstName !== null && person.firstName !== undefined) {
name = person.firstName;
} else {
name = 'John';
}
Now you may be wondering what the difference is between ??
and the logical OR operator||
since they can technically be used interchangeably. However, there is one key difference between the two. As my example shows, nullish coalescing only checks for null
and undefined
rather than other falsy values (like 0, ‘’, false, etc.). Let’s look at an example.
function doSomething(value: number) {
const variable = value || 5;
return variable * 2;
}
If we were to pass in 0 to this function we would get 10 as an output. This can be a bug since we may only want to check if the value is null or undefined. Let’s change this to use nullish coalescing.
function doSomething(value: number) {
const variable = value ?? 5;
return variable * 2;
}
A simple change makes a big difference because if we were to pass in 0 now we would get 0 as an output since nullish coalescing only checks for null and undefined.
Hopefully, you guys find some use for nullish coalescing in your code and are able to make use of this nifty new feature!
If you’ve enjoyed this article I’d love for you to join my mailing list where I send out additional tips & tricks!
If you found this article helpful, interesting, or entertaining buy me a coffee to help me continue to put out content!