Why you should consider using Svelte
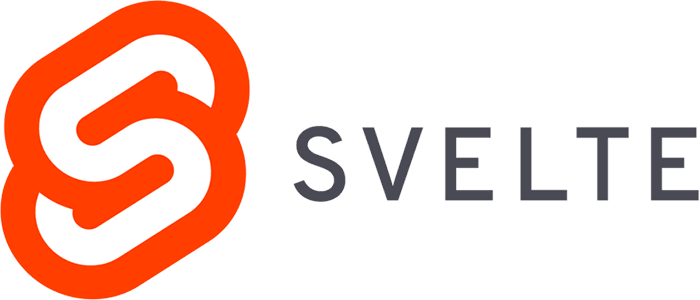
This week I decided to give Svelte a go and build an app. I wanted to answer a few questions, namely, what is it? What does it bring to the table? Should you consider it for your next project? I’ve never used Svelte before so this will be a learning experience for me as well. This will be part 1 in a 2 part series of my journey into learning Svelte. I wanted to do this all in 1 post but I feel like I have too much to write about and I’d rather break it up a bit.
A brief history
Before we dive right in I wanted to get a little background on Svelte. We’ll start with its initial release back in November of 2016. A guy names Rich Harris came up with this slick front-end compiler. If the name Rich Harris sounds familiar to you, that would be because he had also created Reactive.js earlier which is the predecessor to Svelte. The main goal, it seems, was to create something that allows you to write pure reactive code in JavaScript. Some of you may be wondering, what is reactive programming?
I’ll try to keep it brief so as not to get too off-topic. Reactive programming is basically a program that reacts to events over time. That basically means that your program reacts to a series of events that happen over a period of time. As you can imagine reactive programming mainly deals with quite a lot of asynchronous data. Both Reactive.js and Svelte are designed to enable you to write better reactive front-ends. The Svelte developers maintain that Svelte allows you to write truly reactive code in plain old JavaScript.
Ok so now we know that Svelte is based on Reactive.js and that one of its goals is to allow better reactive front-ends. Now we need to talk about Svelte’s bread and butter: the compiler. Unlike other libraries and frameworks, Svelte’s main concept is to shift much of the bulk of the work your app does from the browser to the compiler. Svelte's core idea is that it will compiler your code down to nothing more than vanilla JS with absolutely no framework references. This would allow your final bundle to be extremely small and your app would start up extremely fast. If you want to learn more about it, I would highly recommend reading Rethinking Reactivity on the Svelte website.
[embed]https://svelte.dev/blog/svelte-3-rethinking-reactivity[/embed]
So with that small history lesson out of that way I’m super excited to get into it!
Getting started & first impressions
Oftentimes people don’t know how to start a journey into new technology. I decided to start on the Svelte website where they have nice easy links to the documentation, code examples, and a handy-dandy tutorial. I decided that the best way was to just jump right in with their interactive tutorial.
[embed]https://svelte.dev/blog/svelte-3-rethinking-reactivity[/embed]
During the tutorial, I discovered how simple writing code in Svelte was. I would encourage you to do some of the tutorials yourself because it’s really quite fascinating. It takes literally no time to get up and running with Svelte and your components are super simple. I really like how, unlike React, it doesn’t use JSX or TSX. I find those kinds of things to be really cumbersome and clunky. Instead, you can keep your styles, code, and markup in the same file but there are degrees of separation. I also really like the fact that none of the styles from one component leak into another component I might want to use.
Things I liked
One of the features I really like is that Svelte warns you about accessibility issues. With how many inaccessible websites there are I like the fact that this helps me make my app accessible to such a wide user base. You see companies like Toys “R” Us and Dominos getting sued (quite rightfully so) because their sites/apps can’t be used by people with vision impairments. I think it was Toys “R” Us that actually used images to display blocks of text, which is just plain awful (please never do this).
One thing I found very interesting was their reactive syntax. If you didn’t go through the tutorial here is an example:
<script>
let count = 0;
$: console.log(`the count is ${count}`);
$: double = count * 2;
function handleClick() {
count += 1;
}
</script>
<button on:click={handleClick}>
Clicked {count} {count === 1 ? 'time' : 'times'}
</button>
<p>{count} doubled is {double}</p>
The odd $: syntax basically tells Svelte that whenever your component state changes re-run this thing. So every time we click the button it will re-run count * 2 as well as console logging the current value of count. What happens when we replace $: with let or remove it? Absolutely nothing, it won’t work the same. the console log will run once as well as count * 2. It will never be re-run. I can already tell that this will bring significant performance enhancements since the whole thing doesn’t get re-rendered on every state change.
One thing I really liked was their markup logic syntax. If you wanted to add an if/else block Svelte lets you chain if/else blocks like you would in any programming language. I also really liked the way they handle dynamic lists. Let’s look at some examples:
https://gist.github.com/shadow1349/bb8a160762b764e4539b3bf196899433
This is taken right out of the Svelte tutorial. If you didn’t go through the tutorial let me explain one thing about exports. When you declare “export let name” you can now pass name into that component as a prop.
Now you can see an example of toggling something in the view based on a variable, like if the user is logged in or not. I really like the way we can chain those if-else statements and the syntax is simple which makes it easy to follow.
Let’s also look at how it does change detection for lists like in the {# each} block. For one, the syntax for all of these things is basically going to be the same which makes writing the code easier and more predictable. Another thing is that when you do (thing.id) at the end you tell Svelte that thing.id is a key we should follow, not unlike passing a key to a prop in React or using a trackBy function in Angular except that it’s way more simple in Svelte.
Let’s talk about async stuff. For some background on my preferences, I really like Angular and RxJS. I feel like they make async things way easier. Specifically, I like the fact that in Angular I can easily await a promise or an observable with a pipe like so:
<p>{{someAsyncValue | async}}</p>
After looking at Svelte’s implementation I REALLY like how it works. Here’s their example:
<script>
async function getRandomNumber() {
const res = await fetch(`tutorial/random-number`);
const text = await res.text();
if (res.ok) {
return text;
} else {
throw new Error(text);
}
}
let promise = getRandomNumber();
function handleClick() {
promise = getRandomNumber();
}
</script>
<button on:click={handleClick}>
generate random number
</button>
<!-- replace this element -->
{#await promise}
<p>loading...</p>
{:then number}
<p> the number is {number} </p>
{:catch error}
<p> {error.message} </p>
{/await}
Just like making an if/else block you can basically chain your promise in the markup. I love this because it makes showing a loading indicator super simple as well. With Angular to get the same effect, it’s not too bad, but you have to know your way around observables and there’s a lot more work involved. In Svelte it’s super simple and right to the point. Not to mention it’s also easy to read. You can figure out what’s going on without knowing anything about Svelte and that’s awesome.
There’s so much more I want to include here but I don’t want to make this post a whole book, so at this point, I would highly encourage you to go through the tutorial on their website (sorry if I didn’t list your favorite thing about Svelte). I guarantee whether you’re coming from vanilla JavaScript, Angular, Vue, or React you’ll be pleasantly surprised at how much you like Svelte and, like me, pretty impressed by it overall.
Things I didn’t like
So far I feel like I’ve been gushing a bit (and for good reason), so I want to hit on some negatives I can see because it’s not all sunshine and rainbows. One big old negative is the fact that Svelte does not perform any kind of sanitization of dynamically inserted markup/code. Let’s look at an example:
<script>
let string = `this string contains some <strong>HTML!!!</strong>`;
</script>
<p>{@html string}</p>
Using the @html tag I can basically ensure that any kind of HTML tag will be run in the browser with absolutely no sanitization. In their tutorial, they even say that you’ll need to sanitize it yourself to avoid the possibility of a Cross-Site Scripting (XSS) attack. I don’t like this because if someone is starting with Svelte and they don’t really understand the risk involved here they can create big fat vulnerabilities in their code and there’s nothing to stop that from happening.
The other one kind of has to do with how reactivity works. I would say I feel more indifferent about this one. I went over how their reactivity works briefly with the $: symbol but there is a small catch. This needs an assignment to work:
<script>
let numbers = [1, 2, 3, 4];
function addNumber() {
numbers.push(numbers.length + 1);
numbers = number; // assigning so $: sum will re-run
}
$: sum = numbers.reduce((t, n) => t + n, 0);
</script>
<p>{numbers.join(' + ')} = {sum}</p>
<button on:click={addNumber}>
Add a number
</button>
This can seem somewhat clunky and is really the first solution one may think of and is absolutely a valid way to get it to re-run (although not the best way). The reason I’m kind of indifferent about this is that, if you’re not lazy, it forces you to think of better ways to do it which is a good thing. For example:
numbers = [...numbers, numbers.length + 1];
Their tutorial details other ways you can get around randomly assigning a variable to itself as well but I’m sure you can think of them yourself. On one hand, I like the fact that this forces me to think a little bit more, but I also don’t like the fact that this could potentially lead someone into doing something really stupid but still valid.
The one last thing I didn’t really like is the fact that to get this all working in my local development environment I need to use webpack or rollup. While the implementations of both are officially maintained and there’s lots of documentation and examples one thing I absolutely HATE doing is writing/figuring out config. This is probably more of a personal thing, but I just want to focus on my product. I don’t want to spend any of my time writing boilerplate configs. I would prefer that all be done for me since they’re usually pretty standard. If you’re a person who doesn’t mind doing that sort of thing this may not apply to you, but that’s my take on it at least. Is it lazy? Maybe. Personally I feel like I’d rather work on my project that work on the config for my project.
I’m going to kind of retract that last statement. So there is a thing called SvelteKit that basically does everything I want. It will create a boilerplate Svelte project with some base config that you can alter later but it’ll get you up and running quickly.
Creating my own project
I really want to give Svelte a proper spin around the block, but I fear this is getting a touch too long. I’m going to turn this into a two part post where the second part is a breakdown of using Svelte to create a todo list app. With that being said I hope this has inspired you to take a look into Svelte for your next front-end application.
If you’ve enjoyed this article I’d love for you to join my mailing list where I send out additional tips & tricks!
If you found this article helpful, interesting, or entertaining buy me a coffee to help me continue to put out content!