Angular Project Structure
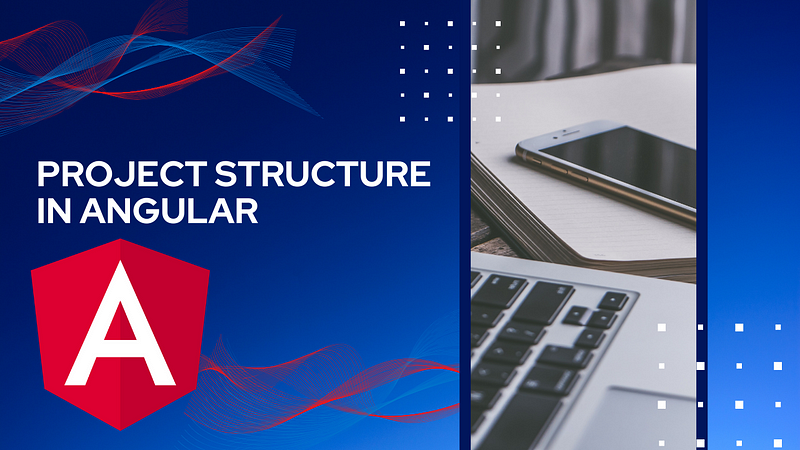
I've talked a lot about how Angular has a standard way of doing everything. However, that doesn't mean everything is taken care of for you. You are still at the helm of the project, and there are decisions you still need to make. One of those decisions is how to structure your projects. Angular has many elements you can use, like modules, components, services, directives, etc. You need to make some decisions about where those components of your application will go. I think this is one of those decisions we all have to make regardless of what framework/library or even what language you're using. In this article, I wanted to take you through how I structure my projects, and hopefully, that will help some of you out as well.
The Basics
Let's first look at the basics. Once we create a brand new Angular project, what will it look like? For reference, I'm creating a new Angular project with routing. If you choose no to the routing option, you will not have the app-routing.module.ts.
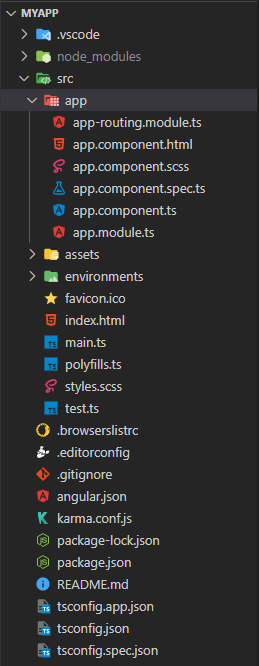
The meat of the application is stored within the app folder. The assets folder is an empty folder, and the environments folder just has the config for each environment you want. We won't really be looking at those today. Right away, we can see a pattern start to emerge. We usually keep components and their module within the same directory. Generally, if you're going to make a new component, you would include it in the app module or create a new module for it.
Modules and Components
So let's say our application will share a bunch of components that repeat throughout the whole app. I would store those in a separate folder called "components" or something like that.
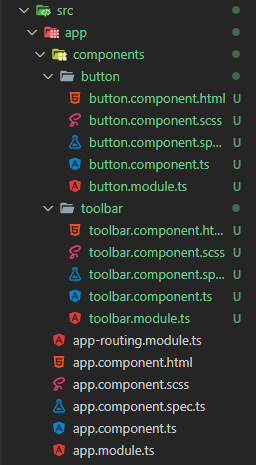
This seems kind of simple, but there is a little bit of nuance here. First of all, we can see that each component has its own module. There are a few ways you could do this. You could create a module called components and include all of the Angular components in that one module. The other option is to just have a folder called components; each component will have its own module. This is the preferable approach, in my opinion. If you were to have one supermodule with a bunch of components, every time you import that into another module to use one of the components, you're also importing all the other components. It can make your app a lot less efficient. It can also make your app a lot less readable. It's easier to follow your app if you're importing individual components with a logical name (like "button" or something like that).
You may have also noticed that these do not contain the same routing modules as the app module. The reason is that these are not meant to be individual routes but re-usable pieces of a larger application.
Routes
I generally tend to keep my routes as simple as I can. I store all my routes inside a folder called "routes." Feel free to use whatever terminology makes sense to you (some people like "pages"), but that's the easiest thing for me to understand when I look at the project at a high level.
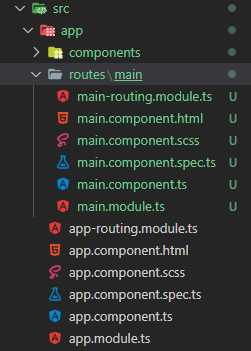
Sub Routes
It's also pretty standard to include sub-routes inside of your project. Let's see what that would look like.
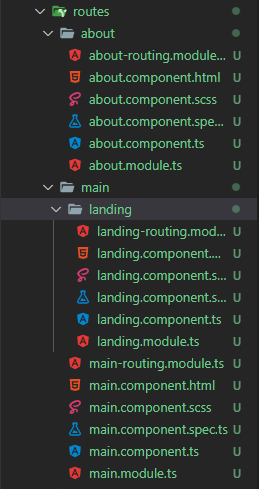
My goal for my projects is to be able to read the project structure like a book. Everything should be plainly spelled out and easy to follow. I get annoyed when I see developers naming things in a really convoluted way. Let's have a look at our project from a high level at this point.
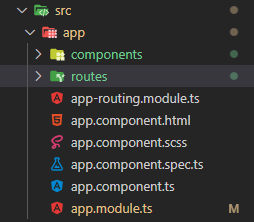
Where would you look if you were asked to modify the title of the about page? You'd immediately know to go into the routes folder where you'd see about and find the correct component. It's as easy as turning to the book's next page.
Services
If you're new to the Angular world, services are where you will do things like make API calls. I generally tend to stick with the Angular terminology and call the directory "services." One note, when you create an Angular service using the CLI it will not create its own directory like it does when you create a component or module. I'm not sure why it does that, but that's what it does. So when you create a service, I generally tend to do something like this.
ng g s services/api/api
That will keep everything nicely in its place. Now you can find all your services neatly tucked away in the services directory. You'll know where to go when you want to make an API call.
General Rule
How you structure your project is really up to you and how to make the best of the project you're working on. What I've shared with you today is what has worked best for me. The general rule of thumb I follow is to follow a naming convention that you can understand for your folders. For example, if "services" doesn't make sense to you, call it "backend" or "api" instead; just make sure that all the Angular services that call your APIs stay in that directory.
Hopefully, this has helped you make some good project structure decisions for your next Angular project!
If you've enjoyed this article, I'd love for you to join my mailing list, where I send out additional tips, tricks, and newsletters!