TypeScript Tip of the Week — Const Assertions using `as const`
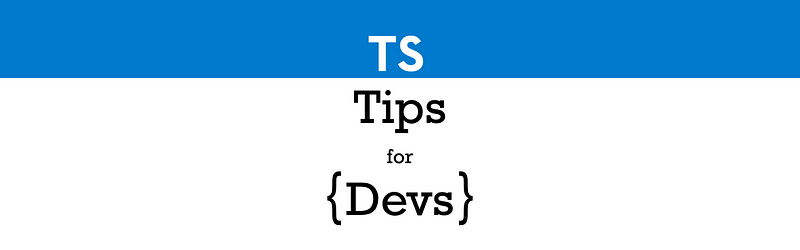
Hey guys, welcome back to another TypeScript Tip! I was on Twitter and came across this awesome TypeScript tip.
[embed]https://twitter.com/sulco/status/1219982511180263424[/embed]
Since Tomek has provided an excellent example of how to use as const
to make your entire object literal immutable, we’re going to focus on why you might want to use this in your code and there are several reasons.
Immutability Provides Predictability
This kind of rhymes so it should be easy to remember. Basically, if you have an object that can’t change then you always know what the values of it are going to be. This becomes useful for things like environment variables, a specific set of settings you may need, or something like conversion charts. An example of this might be converting lbs to kgs.
const conversion = {
lb: 0.45,
kg: 2.2
} as const
Now we can tell 1 lb is equal to roughly 0.45 kg and 1 kg is equal to roughly 2.2 lb. These values must remain constant and should not be changed, which is a perfect way to use as const
. Since it will also make nested objects immutable we can have this object be as complex as we want.
Performance Gains
While this may seem a little picky, immutable objects do provide some performance gains since they don’t need to be changed. This takes some strain off of the host memory. This may be a small gain, but it is an advantage of immutable objects. One thing to be aware of is that if a value in our immutable object needs to change for whatever reason we do have to restart the code, which can be a little bit of a pain, so be careful what you’re making immutable.
Cleaner code and Easy to Test
Since immutable objects are predictable they are both easy to test and easy to read. 90% of our job is maintaining code so it helps when it’s easy to read. Part of that responsibility is testing the code we’re maintaining/writing. When the code is predictable it’s also easy to test which makes out lives very easy.
Last Thoughts
If done correctly, immutable objects can provide a great benefit to your code. TypeScript allows us to easily make complex object literals immutable with the as const
assertion.
I wanted to thank Tomek for posting this tip and allowing me to feature it in this article. Immutable objects are an awesome tool in your belt and using as const
makes object literals immutable with minimal effort. I hope you guys enjoyed this article!
If you’ve enjoyed this article I’d love for you to join my mailing list where I send out additional tips & tricks!
If you found this article helpful, interesting, or entertaining buy me a coffee to help me continue to put out content!