TypeScript Tip of the Week — Optional Chaining
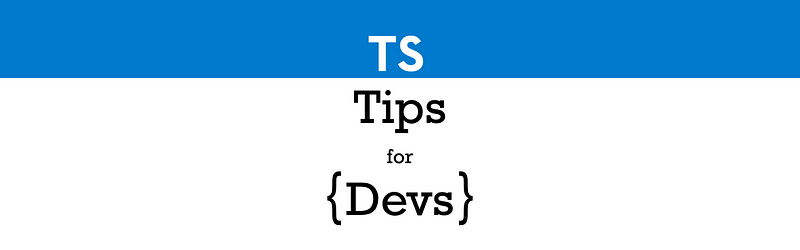
Optional chaining is another fantastic feature of TypeScript 3.7 that we should all be using. As usual, let’s have a look at an example of what optional chaining is.
interface PersonData {
firstName: string;
}
interface Person {
data?: PersonData;
}
const person: Person = {
data: {
firstName: "Sam"
}
};
const name = person?.data?.firstName;
What this will do is check each property to make sure we don’t have null or undefined anywhere in the chain. If person
, data
, or firstName
are null or undefined name resolve to be undefined
. Before we could use optional chaining if part of this chain was null or undefined our app would throw an error. Let’s look at another example.
const person: Person = {
data: null
};
const name = person?.data?.firstName;
console.log(name); // undefined
In this example, we can see that the data property of Person in null. As you can see, rather our program throwing an error, name
will be undefined. This can help our code react a little more consistently when we have object chains where a property might be null or undefined.
If you’ve enjoyed this article I’d love for you to join my mailing list where I send out additional tips & tricks!
If you found this article helpful, interesting, or entertaining buy me a coffee to help me continue to put out content!